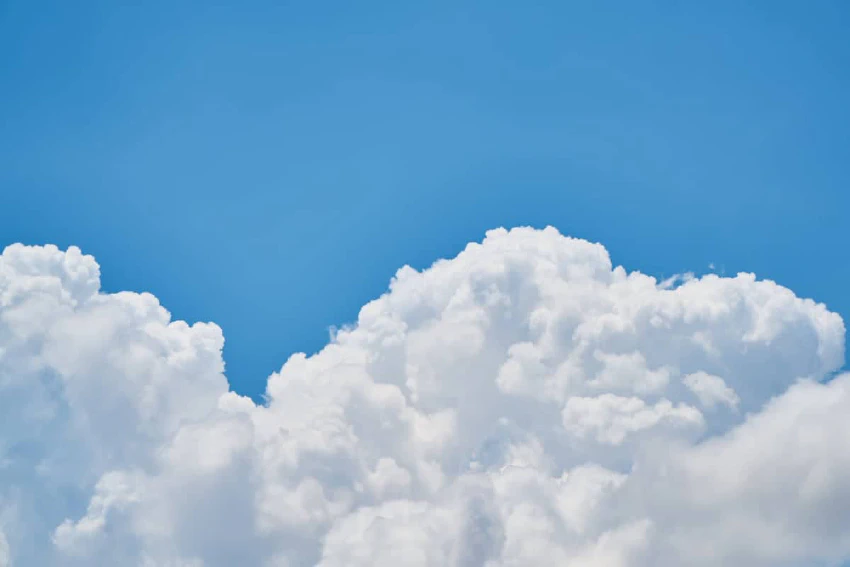
f451 Labs RPI Cloud module
This module has been integrated into the f451 Labs Common module and the GitHub repo will be removed shortly.
Overview
The f451 Labs Cloud module encapsulates the Adafruit IO REST and MQTT clients, as well as the Arduino Cloud client within a single class. Most f451 Labs projects upload to and/or receive data from one or both of these services, and the Cloud
class simplifies these tasks by standardizing ‘send’ and ‘receive’ methods.
The f451 Labs Cloud exports the Cloud
object class, which offers the most commonly used Adafruit IO and Arduino Cloud services as methods. The basic idea is to hide the complexities and differences between various IO clients inside a common object and offer a simplified interface to f451 Labs applications.
This encapsulation also allows us to add, modify, and remove clients and services without much affecting the other f451 Labs applications. Of course, the main benefit is that we can use the same set-up/config process across our applications, most of which interact with one or more cloud services.
Core functions
This module includes the following functions:
- aio_create_feed() — Create a new Adafruit IO feed.
- aio_feed_list() — Get complete list of Adafruit IO feeds.
- aio_feed_info() — Get info/metadata for an Adafruit IO feed.
- aio_delete_feed() — Delete an existing Adafruit IO feed.
- aio_send_data() — Send data to an existing Adafruit IO feed.
- aio_receive_data() — Receive data from an existing Adafruit IO feed.
Please refer to the GitHub repo for source code and documentation.
Dependencies
This module depends on the following libraries:
How to use
Using the module is straightforward. Simply import
it into your code and instantiate an Cloud
object which you can then use throughout your code.
# Import f451 Labs Cloud
from f451_cloud.cloud import Cloud
# Initialize 'Cloud'
myCloud = Cloud(
AIO_ID = "<ADAFRUIT IO USERNAME>",
AIO_KEY = "<ADAFRUIT IO KEY>"
)
# Create an Adafruit IO feed
feed = myCloud.aio_create_feed('my-new-feed')
# Upload data to Adafruit IO feed
asyncio.run(myCloud.aio_send_data(feed.key, randint(1, 100)))
# Receiving latest data from Adafruit IO feed
data = asyncio.run(myCloud.aio_receive_data(feed.key, True))
# Adafruit IO returns data in form of 'namedtuple' and we can
# use the '_asdict()' method to convert it to regular 'dict'.
# We then pass the 'dict' to 'json.dumps()' to prettify before
# we print out the whole structure.
pretty = json.dumps(data._asdict(), indent=4, sort_keys=True)
print(pretty)